How to add Notification Center support to your website or app
Originally introduced last year in iOS 5, Notification Center is one of the more useful new features in OS X Mountain Lion. What’s really nice is that the ability to show notification banners isn’t limited to native applications; both Safari and Chrome allow websites to show alerts in Notification Center as well.
This is a quick and straightforward guide to adding Notification Center support to your website or web app.
Step 1: Request permission
Your web app can’t show notifications unless the user has explicitly granted it permission to do so. In Safari, permission is requested using a modal sheet dialog, while Chrome displays a banner at the top of the page.
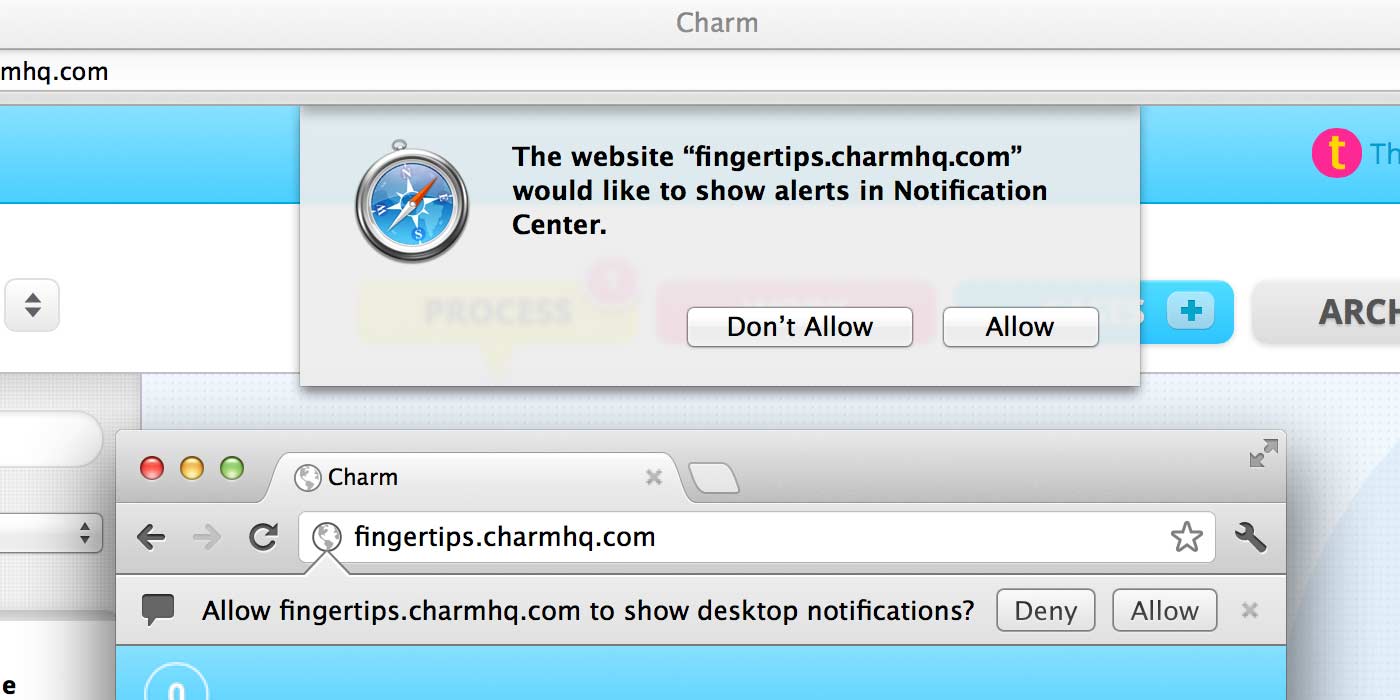
Safari and Chrome requesting permission.
In Safari, users can manage notification permissions in the Notifications Preferences tab:
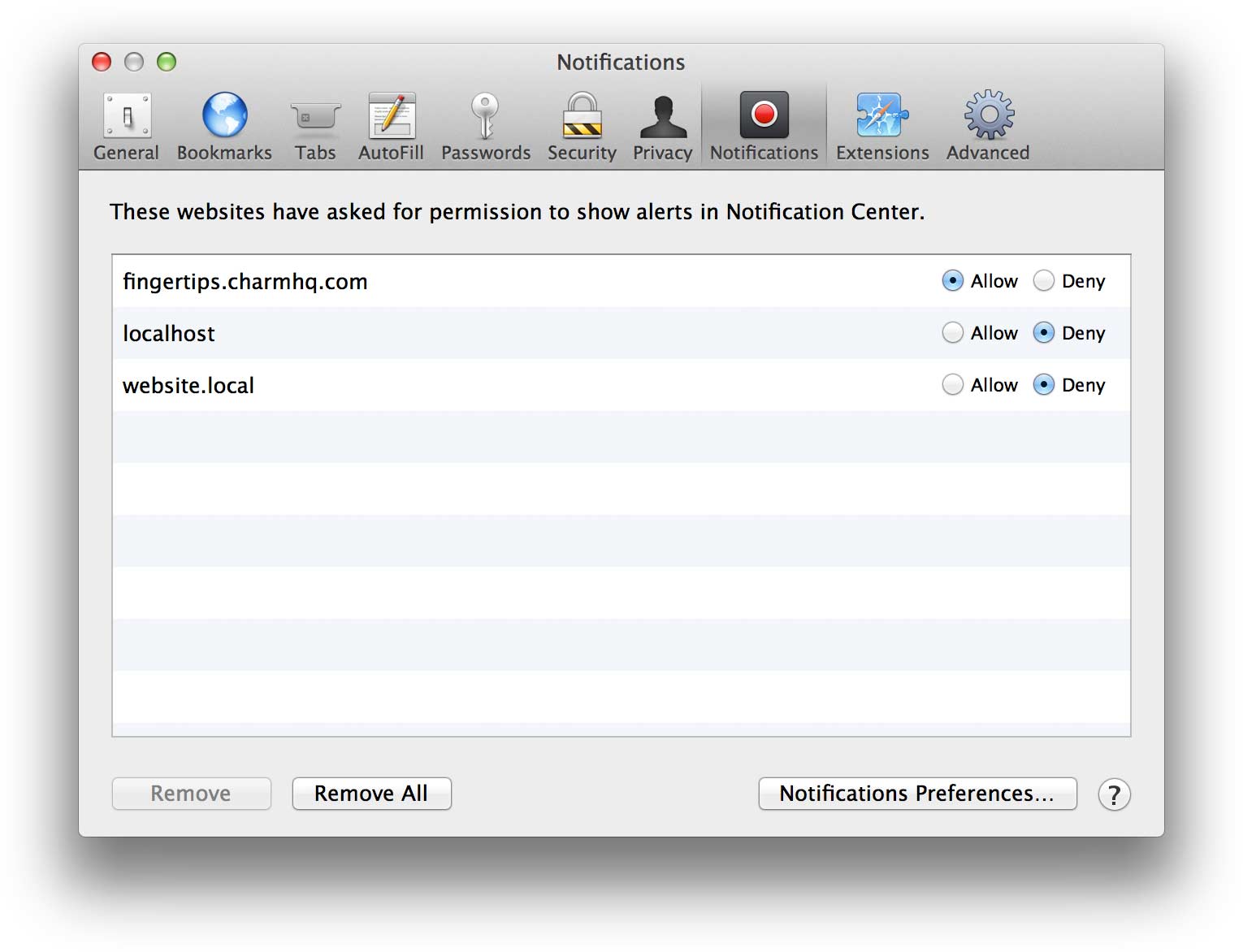
In Safari, the Notifications Preferences are easy to find.
In Chrome, users have to click “Show advanced settings…”, click the “Content Settings…” button under “Privacy”, scroll down to “Notifications” and then click the “Manage exceptions…” button:
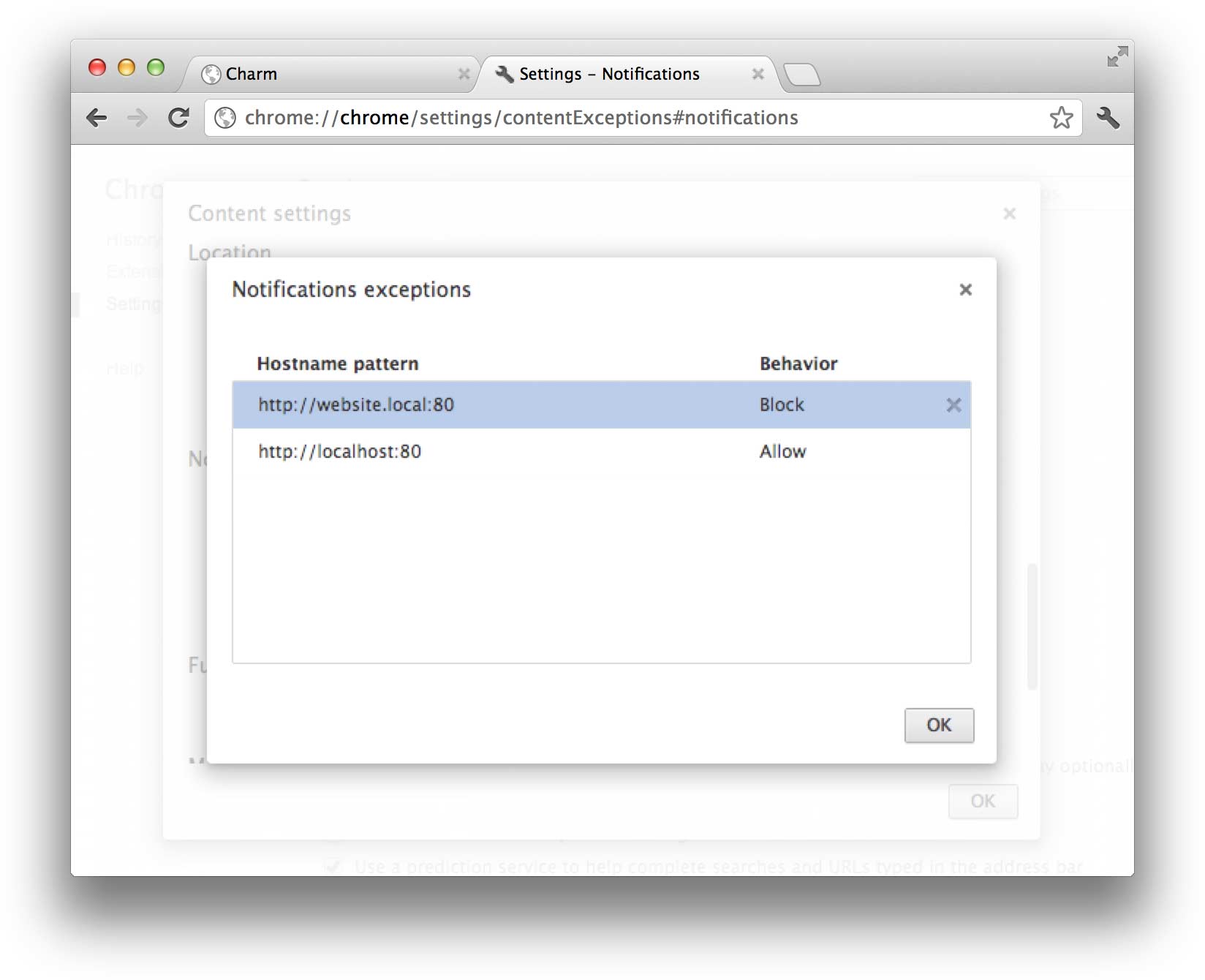
In Chrome, not so much.
There are a few security-related limitation you need to keep in mind when you’re implementing this process:
You can only request permission as the result of a direct user interaction such as clicking a link or a button.
Once you’ve requested permission to show notifications and the user has either allowed or denied, there’s no way you can request permission again. The user can however change or remove the notification setting for your site in the browser preferences.
One other thing to keep in mind is that Safari does not first require permission when it’s showing a HTML file opened from disk. So, make sure you’re testing on a local webserver during development.
The first to do is detect whether notification support is available:
if ('webkitNotifications' in window) {
// Notification support is available
}
Then, call webkitNotifications.checkPermission()
to get your current permission status. The result will be 0
when the user has already granted you permission to show notifications earlier, 1
when you haven’t yet requested permission, and 2
when the user has denied permission.
Only when the permission check returns a 1
should you show an interface element for the user to enable notifications. Call webkitNotifications.requestPermission()
when this interface element has been activated. Requesting permission is asynchronous, so you’ll need to include a callback to handle the result.
$('#request button').on('click', function() {
webkitNotifications.requestPermission(function() {
if (webkitNotifications.checkPermission() == 0) {
// Your user granted you permission to show notifications
}
})
})
Step 2: Show notifications
When you have permission (webkitNotifications.checkPermission()
should return 0
), you can create a notification object with a title and a body:
var notification = webkitNotifications.createNotification(null, 'Hi there', 'I’m notifying you about something that happened just now.');
You probably want to add a callback handler for when the user activates the notification (you can also set handlers for display
, close
, and error
events):
notification.onclick = function() {
// Show the item you notified your user about
}
And finally, you can show the notification with:
notification.show()
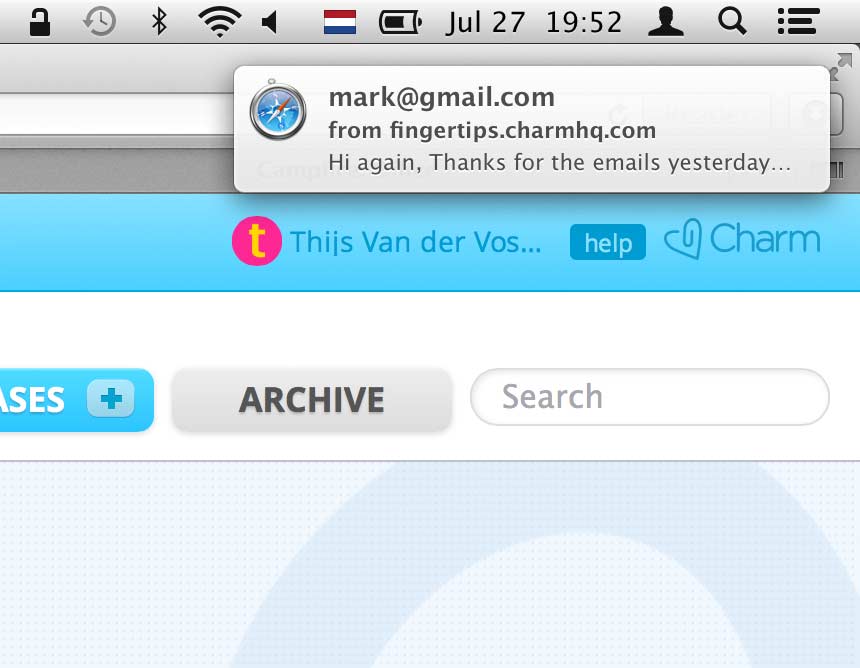
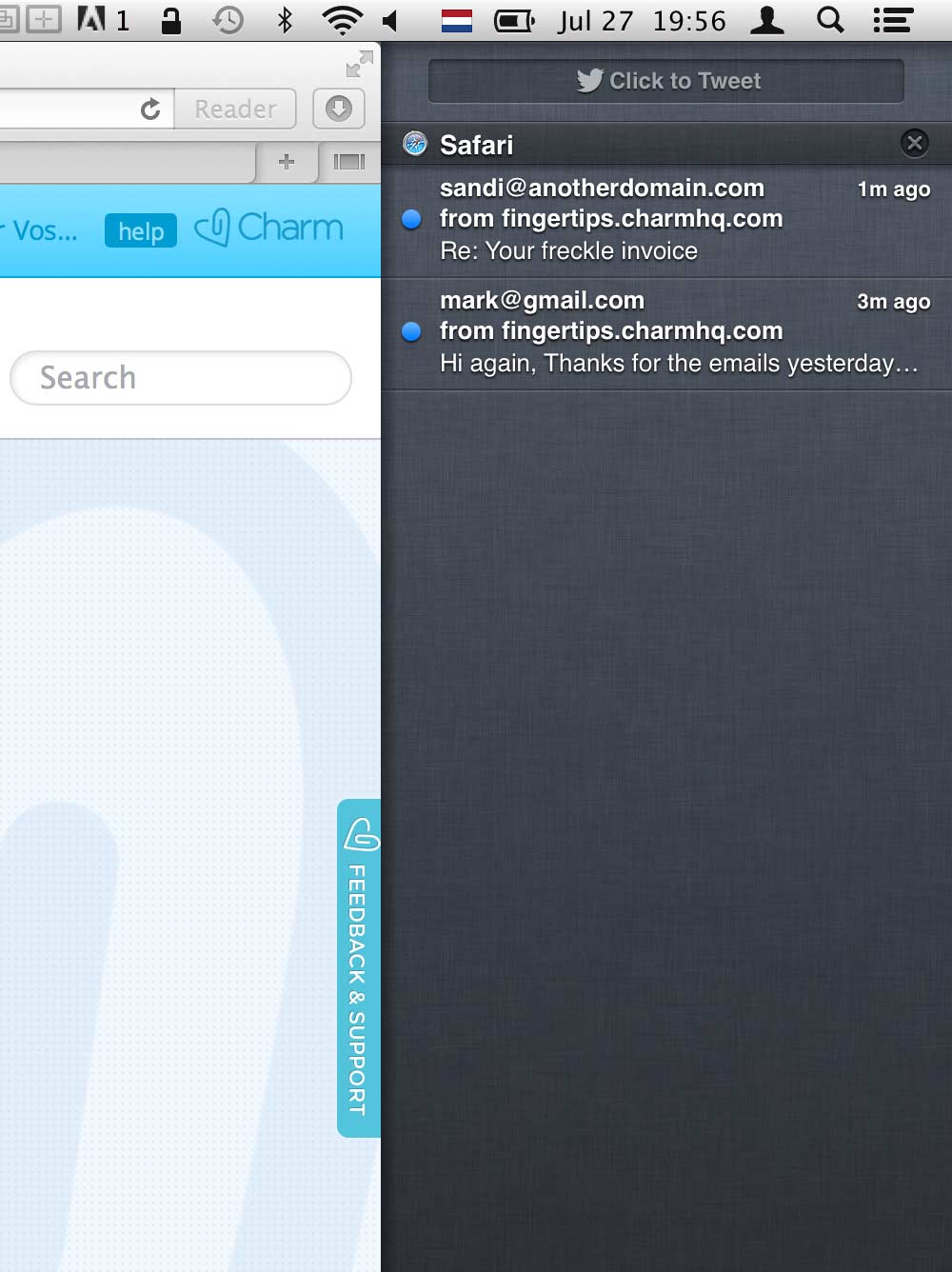
We’re currently consulting on Charm. Adding support for Notification Center took a little over an hour total.
That’s pretty much all there is to it. For more information, see the original Chromium draft specifications, but note that specifying your own icon image and the HTML notification type is not supported on Mountain Lion.
Demo
Here’s a simple but complete Notification Center demo:
View the source of this page to see how the demo is implemented.